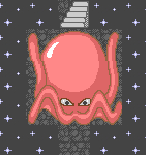 |
【程序】 // regtest.cpp : 定義控制台應用程式的入口點。 //
#include "stdafx.h" #include <iostream> #include <Windows.h>
using namespace std;
int _tmain(int argc, _TCHAR* argv[]) { HKEY hkey; RegOpenKeyEx(HKEY_CURRENT_USER, TEXT("Software\\Microsoft\\Internet Explorer\\Suggested Sites"), NULL, KEY_READ, &hkey);
DWORD cValues; RegQueryInfoKey(hkey, NULL, NULL, NULL, NULL, NULL, NULL, &cValues, NULL, NULL, NULL, NULL); cout << "該鍵下共有" << cValues << "個值 (不含默認值)" << endl;
DWORD cbData; RegGetValueA(hkey, NULL, "SlicePath", RRF_RT_REG_SZ, NULL, NULL, &cbData); cout << "讀取字符串時應該分配的內存空間大小為: " << cbData << "字節" << endl;
char *szValue = (char *)malloc(cbData); // 注意: cbData的單位是字節 RegGetValueA(hkey, NULL, "SlicePath", RRF_RT_REG_SZ, NULL, szValue, &cbData); cout << szValue << endl; // 由於在控制台中輸出寬字符串比較麻煩,所以這裏為了簡單起見用了ANSI版本 cout << "該字符串的實際長度為: " << strlen(szValue) << endl; free(szValue); RegCloseKey(hkey); system("pause"); return 0; } 【輸出】 該鍵下共有10個值 (不含默認值) 讀取字符串時應該分配的內存空間大小為: 47位元組 C:\Users\Octopus\Favorites\Links\建議網站.url 該字符串的實際長度為: 45 請按任意鍵繼續. . .
|
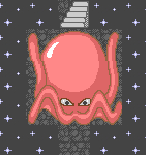 |
這裏要注意的是,由於cbData的單位為字節而非數組的元素個數,所以在用Unicode版本的API函數時要特別注意。最好用C語言的malloc函數分配空間,不要用new WCHAR[cbData],因為這樣的話實際上就分配了cbData * sizeof(WCHAR)字節的內存,造成浪費。
|
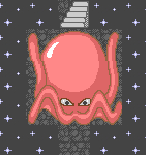 |
在註冊表中寫入字符串非常簡單: // RegTest2.cpp : 定義控制台應用程式的入口點。 //
#include "stdafx.h" #include <Windows.h>
int _tmain(int argc, _TCHAR* argv[]) { // 打開註冊表鍵 // 如果鍵不存在,則自動創建(這個非常常用) HKEY hkey; RegCreateKey(HKEY_CURRENT_USER, TEXT("Software\\應用程式嚮導生成的本地應用程式\\test"), &hkey);
TCHAR szValue[] = TEXT("這是一個中文字符串"); RegSetValueEx(hkey, NULL, NULL, REG_SZ, (BYTE *)szValue, sizeof(szValue));
TCHAR szValue2[] = TEXT("簡體中文abc"); RegSetValueEx(hkey, TEXT("string"), NULL, REG_SZ, (BYTE *)szValue2, sizeof(szValue2)); DWORD dwValue = 0x12345678; RegSetValueEx(hkey, TEXT("number"), NULL, REG_DWORD, (BYTE *)&dwValue, sizeof(DWORD));
RegCloseKey(hkey); return 0; }
|
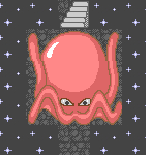 |
可以將1樓所述的方法做成一個返回std::string字符串的函數: // regtest.cpp : 定義控制台應用程式的入口點。 //
#include "stdafx.h" #include <iostream> #include <string> #include <Windows.h>
using namespace std;
string RegReadStringA(HKEY hkey, char *szSubKey) { DWORD cbData; RegGetValueA(hkey, NULL, szSubKey, RRF_RT_REG_SZ, NULL, NULL, &cbData); char *szData = new char[cbData]; RegGetValueA(hkey, NULL, szSubKey, RRF_RT_REG_SZ, NULL, szData, &cbData); string str(szData); delete[] szData; return str; }
int _tmain(int argc, _TCHAR* argv[]) { HKEY hkey; RegOpenKeyEx(HKEY_CURRENT_USER, TEXT("Software\\Microsoft\\Internet Explorer\\Suggested Sites"), NULL, KEY_READ, &hkey);
string value = RegReadStringA(hkey, "SlicePath"); cout << value << endl; RegCloseKey(hkey); system("pause"); return 0; }
|
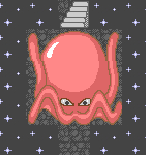 |
對應的寬字符串版本: // regtest.cpp : 定義控制台應用程式的入口點。 //
#include "stdafx.h" #include <string> #include <Windows.h>
using namespace std;
wstring RegReadStringW(HKEY hkey, wchar_t *szSubKey) { DWORD cbData; RegGetValueW(hkey, NULL, szSubKey, RRF_RT_REG_SZ, NULL, NULL, &cbData); wchar_t *szData = (wchar_t *)malloc(cbData); RegGetValueW(hkey, NULL, szSubKey, RRF_RT_REG_SZ, NULL, szData, &cbData); wstring str(szData); free(szData); return str; }
int _tmain(int argc, _TCHAR* argv[]) { HKEY hkey; RegOpenKeyEx(HKEY_CURRENT_USER, TEXT("Software\\Microsoft\\Internet Explorer\\Suggested Sites"), NULL, KEY_READ, &hkey);
wstring value = RegReadStringW(hkey, L"SlicePath"); MessageBoxW(NULL, value.c_str(), L"字符串", MB_ICONINFORMATION); RegCloseKey(hkey); return 0; }
|
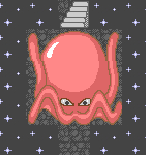 |
如果要獲取一個鍵下的所有字符串值,可以先通過RegQueryInfoKey函數獲取最長字符串值的長度,然後一次性分配空間,提高效率。
|