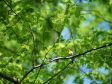 |
11樓
蓝晶の骑士
2011-2-10 23:36
for i in 0..2 # For each layer for j in [0, (($height / 2) - (($height * 60) / @pitch) + @oy) / $strip_size].max.to_i..[@sprites[i].size - 1, (@oy + $height) / $strip_size].min.to_i # For each strip within the visible screen, update OX/Y @sprites[i][j].x = $width / 2 @sprites[i][j].y = j * $strip_size - @oy unless @pitch == 0 # Apply X Zoom @sprites[i][j].zoom_x = (@sprites[i][j].y - $height / 2) * (@pitch / ($height * 25)) + 1 if $curve # Zoom Y values same as X, and compensate @sprites[i][j].zoom_y = @sprites[i][j].zoom_x @sprites[i][j].y += $strip_size * (1 - @sprites[i][j].zoom_y) * ((1 - @sprites[i][j].zoom_y) / (2 * ((@pitch / 100) / ($height / ($strip_size * 2)))) + 0.5) end end @sprites[i][j].ox = @ox + $width / 2 # Add plus_y value; used in airship script @sprites[i][j].y += @plus_y end end end #----------------------------------------------------------------------------- def dispose # Dispose all sprites for i in 0..2 for j in @sprites[i] j.bitmap.dispose j.dispose end end for i in @bitmaps i.dispose end @tileset.dispose for i in 0..6 @autotiles[i].dispose end @disposed = true end #----------------------------------------------------------------------------- def disposed? return @disposed end #----------------------------------------------------------------------------- def draw # Draw each individual position by XY value for x in 0...@map_data.xsize for y in 0...@map_data.ysize draw_position(x, y) end end for i in 0..2 # For each priority for j in 0..@sprites[i].size - 1 # For each horizontal strip, transfer the bitmap appropriately @sprites[i][j].bitmap.blt(0, 0, @bitmaps[i], Rect.new(0, j * $strip_size, $game_map.width * 32, $strip_size * 2)) end end end #----------------------------------------------------------------------------- def draw_position(x, y) for layer in 0..2 pos = @map_data[x, y, layer] @priorities[pos] = 2 if @priorities[pos] > 2 # Round priorities down to 2 if pos >= 384 # If it is a tile # src_rect = 32x32 Rect on the tileset for source bitmap src_rect = Rect.new(((pos-384)%8)*32, ((pos-384)/8)*32, 32, 32) # Transfer source bitmap on the tileset to the current map tile @bitmaps[@priorities[pos]].blt(x * 32, y * 32, @tileset, src_rect) elsif pos >= 48 and pos < 384 # If it is an autotile id = pos / 48 - 1 # Which autotile is used (0-6) # plus_x is in development for animated autotiles plus_x = 0 #((@anim / 4) % (@autotiles[id].width / 96)) * 96 for corner in 0..3 h = 4 * (pos % 48) + corner # Used to access INDEX # src_rect = 16x16 Rect on the autotile for source bitmap src_rect = Rect.new((INDEX[h]%6)*16+plus_x, (INDEX[h]/6)*16, 16, 16) # Transfer source bitmap on the autotile to the current 16x16 tile @bitmaps[@priorities[pos]].blt(x*32+X[corner]*16, y*32+Y[corner]*16, @autotiles[id], src_rect) end end end end end #=============================================================================== class Game_Map attr_accessor :pitch
|
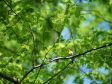 |
12樓
蓝晶の骑士
2011-2-10 23:37
attr_accessor :plus_y #----------------------------------------------------------------------------- alias setup_or :setup def setup(map_id) setup_or(map_id) @pitch = $data_map[$game_map.map_id].pitch @plus_y = 0 end #----------------------------------------------------------------------------- def name return $data_map[@map_id].name end end #=============================================================================== class Sprite_Character < RPG::Sprite attr_accessor :character #----------------------------------------------------------------------------- def initialize(character = nil) super() @character = character update end #----------------------------------------------------------------------------- alias update_or :update def update update_or # Update pitch value, and update zoom values to match @pitch = $data_map[$game_map.map_id].pitch.to_f self.zoom_x = self.zoom_y = ((@character.screen_y - 16) - ($height / 2)) * (@pitch / ($height * 25)) + 1 # Set sprite coordinates. X value is multiplied by zoom value from the center self.x = ($width / 2) + ((@character.screen_x - ($width / 2)) * self.zoom_x) self.y = @character.screen_y # Add Y value for zoom compensation while in curve mode if $curve and @pitch != 0 self.y += (8 * (1 - self.zoom_y) * ((1 - self.zoom_y) / (2 * ((@pitch / 100) / ($height / 16.0))) + 0.5)) end # Add plus_y value; used in airship script self.y += $game_map.plus_y unless @character.is_a?(Game_Player) self.z = @character.screen_z(@ch) - (self.zoom_y < 0.5 ? 1000 : 0) if $data_map[$game_map.map_id].overworld? and @character.is_a?(Game_Player) # Multiply zoom by Overworld factor if self.zoom_x *= $ov_zoom # the map is marked with [OV] and event self.zoom_y *= $ov_zoom # is a Game_Player end end end #=============================================================================== class Spriteset_Map def initialize # Make viewports @viewport1 = Viewport.new(0, 0, 640, 480) @viewport2 = Viewport.new(0, 0, 640, 480) @viewport3 = Viewport.new(0, 0, 640, 480) @viewport2.z = 2000 @viewport3.z = 5000 # Make tilemap @tilemap = Draw_Tilemap.new # Make panorama plane @panorama = Plane.new @panorama.z = -2000 # Make fog plane @fog = Plane.new @fog.z = 3000 # Make character sprites @character_sprites = [] for i in $game_map.events.keys.sort sprite = Sprite_Character.new($game_map.events[i]) @character_sprites.push(sprite) end @character_sprites.push(Sprite_Character.new($game_player)) # Make weather @weather = RPG::Weather.new(@viewport1) # Make picture sprites @picture_sprites = [] for i in 1..50 @picture_sprites.push(Sprite_Picture.new(@viewport2, $game_screen.pictures[i])) end # Make timer sprite @timer_sprite = Sprite_Timer.new # Frame update update end #----------------------------------------------------------------------------- def dispose # Dispose of tilemap @tilemap.dispose # Dispose of panorama plane @panorama.dispose # Dispose of fog plane @fog.dispose # Dispose of character sprites for sprite in @character_sprites sprite.dispose end # Dispose of weather @weather.dispose # Dispose of picture sprites for sprite in @picture_sprites sprite.dispose end # Dispose of timer sprite @timer_sprite.dispose # Dispose of viewports @viewport1.dispose @viewport2.dispose @viewport3.dispose end end
|